The first step is to create the project.
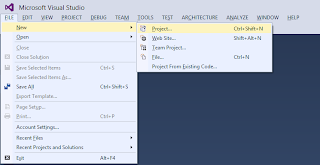
Select a typescript project.

We need to install four packages.
- Angular.js
- JQuery.js
- Typescript definition for Angular.js
- Typescript definition for JQuery.js
- Install-Package angularjs
- Install-Package jQuery
- Install-Package angularjs.TypeScript.DefinitelyTyped
It is possible to specify a specific version of the package on the command line. Without the version specification, Nuget will install the most recent version. Installing the TypeScript definition for angularjs will also install the typescript definition for jQuery.
The next step requires changing the index.html file. Below is the default file.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <title>TypeScript HTML App</title> <link rel="stylesheet" href="app.css" type="text/css" /> <script src="app.js"></script> </head> <body> <h1>TypeScript HTML App</h1> <div id="content"></div> </body> </html>
I am not sure why the default file has a blank line between the DOCTYPE declaration and the HTML declaration. Modify the html element to add the ng-app attribute. Intellisence helps add the definition. Add a value of "DemoApp" for the attribute name. This is the name of the single page application. Angular.js allows multiple applications.
<html lang="en" ng-app="DemoApp">
In the Solution Explorer, open the Scripts folder.
We need to include either the angular.js file or the angular.min.js file as a script. Debugging with the minified version of the JavaScript file is difficult. Grab the angular.js file; drag it under the link element in the index.html file; then drop the file. Visual Studio will insert the link.
<!DOCTYPE html> <html lang="en" ng-app="DemoApp"> <head> <meta charset="utf-8" /> <title>TypeScript HTML App</title> <link rel="stylesheet" href="app.css" type="text/css" /> <script src="Scripts/angular.js"></script> <script src="app.js"></script> </head> <body> <h1>TypeScript HTML App</h1> <div id="content"></div> </body> </html>
Next, modify the app.ts file to initialize Angular.js. We named the application "DemoApp" above when we modified the html element. We need to add a line to the app.ts file to initialize that module. Add the following line to the app.ts file.
var demoAppModule = angular.module('DemoApp');
That declares a variable, demoAppModule, to hold the Angular.js module. The module method takes the name of the application.
Now we have a minimal TypeScript application using Angular.js.
1 comment:
Hi, Great.. Tutorial is just awesome..It is really helpful for a newbie like me.. I am a regular follower of your blog. Really very informative post you shared here. Kindly keep blogging. If anyone wants to become a Front end developer learn from TypeScript Training in Chennai . or learn thru Javascript Online Training from India. Nowadays JavaScript has tons of job opportunities on various vertical industry. ES6 Training in Chennai
Post a Comment